In this article, learn how to manage request routing and how to use the NavLink component to create a navigation links in Blazor apps.
We discuss the three project types available with Blazor 0.5.1, get an understanding of what scenarios to apply them to, and explore what to expect from each. The Blazor WYSIWYG Rich Text Editor works as markdown text editor that provides the best user interface for creating and editing markdown content.
Route templates
The Router component enables routing to Razor components in a Blazor app. The Router component is used in the App
component of Blazor apps.
App.razor
:
Note
With the release of ASP.NET Core 5.0.1 and for any additional 5.x releases, the Router
component includes the PreferExactMatches
parameter set to @true
. For more information, see Migrate from ASP.NET Core 3.1 to 5.0.
When a Razor component (.razor
) with an @page
directive is compiled, the generated component class is provided a RouteAttribute specifying the component's route template.
Blazor Markdown Control
When the app starts, the assembly specified as the Router's AppAssembly
is scanned to gather route information for the app's components that have a RouteAttribute.
At runtime, the RouteView component:
- Receives the RouteData from the Router along with any route parameters.
- Renders the specified component with its layout, including any further nested layouts.
Optionally specify a DefaultLayout parameter with a layout class for components that don't specify a layout with the @layout
directive. The framework's Blazor project templates specify the MainLayout
component (Shared/MainLayout.razor
) as the app's default layout. For more information on layouts, see ASP.NET Core Blazor layouts.
Components support multiple route templates using multiple @page
directives. The following example component loads on requests for /BlazorRoute
and /DifferentBlazorRoute
.
Pages/BlazorRoute.razor
:
Important
For URLs to resolve correctly, the app must include a <base>
tag in its wwwroot/index.html
file (Blazor WebAssembly) or Pages/_Host.cshtml
file (Blazor Server) with the app base path specified in the href
attribute. For more information, see Host and deploy ASP.NET Core Blazor.
Provide custom content when content isn't found
The Router component allows the app to specify custom content if content isn't found for the requested route.
In the App
component, set custom content in the Router component's NotFound template.
App.razor
:
Note
With the release of ASP.NET Core 5.0.1 and for any additional 5.x releases, the Router
component includes the PreferExactMatches
parameter set to @true
. For more information, see Migrate from ASP.NET Core 3.1 to 5.0.
Arbitrary items are supported as content of the <NotFound>
tags, such as other interactive components. To apply a default layout to NotFound content, see ASP.NET Core Blazor layouts.
Route to components from multiple assemblies
Use the AdditionalAssemblies parameter to specify additional assemblies for the Router component to consider when searching for routable components. Additional assemblies are scanned in addition to the assembly specified to AppAssembly
. In the following example, Component1
is a routable component defined in a referenced component class library. The following AdditionalAssemblies example results in routing support for Component1
.
App.razor
:
Note
With the release of ASP.NET Core 5.0.1 and for any additional 5.x releases, the Router
component includes the PreferExactMatches
parameter set to @true
. For more information, see Migrate from ASP.NET Core 3.1 to 5.0.
Route parameters
The router uses route parameters to populate the corresponding component parameters with the same name. Route parameter names are case insensitive. In the following example, the text
parameter assigns the value of the route segment to the component's Text
property. When a request is made for /RouteParameter/amazing
, the <h1>
tag content is rendered as Blazor is amazing!
.
Pages/RouteParameter.razor
:
Optional parameters are supported. In the following example, the text
optional parameter assigns the value of the route segment to the component's Text
property. If the segment isn't present, the value of Text
is set to fantastic
.
Pages/RouteParameter.razor
:
Optional parameters aren't supported. In the following example, two @page
directives are applied. The first directive permits navigation to the component without a parameter. The second directive assigns the {text}
route parameter value to the component's Text
property.
Pages/RouteParameter.razor
:
Use OnParametersSet
instead of OnInitialized{Async}
to permit app navigation to the same component with a different optional parameter value. Based on the preceding example, use OnParametersSet
when the user should be able to navigate from /RouteParameter
to /RouteParameter/amazing
or from /RouteParameter/amazing
to /RouteParameter
:
Route constraints
A route constraint enforces type matching on a route segment to a component.
In the following example, the route to the User
component only matches if:
- An
Id
route segment is present in the request URL. - The
Id
segment is an integer (int
) type.
Pages/User.razor
:
The route constraints shown in the following table are available. For the route constraints that match the invariant culture, see the warning below the table for more information.
Constraint | Example | Example Matches | Invariant culture matching |
---|---|---|---|
bool | {active:bool} | true , FALSE | No |
datetime | {dob:datetime} | 2016-12-31 , 2016-12-31 7:32pm | Yes |
decimal | {price:decimal} | 49.99 , -1,000.01 | Yes |
double | {weight:double} | 1.234 , -1,001.01e8 | Yes |
float | {weight:float} | 1.234 , -1,001.01e8 | Yes |
guid | {id:guid} | CD2C1638-1638-72D5-1638-DEADBEEF1638 , {CD2C1638-1638-72D5-1638-DEADBEEF1638} | No |
int | {id:int} | 123456789 , -123456789 | Yes |
long | {ticks:long} | 123456789 , -123456789 | Yes |
Warning
Route constraints that verify the URL and are converted to a CLR type (such as int
or DateTime) always use the invariant culture. These constraints assume that the URL is non-localizable.
Routing with URLs that contain dots
For hosted Blazor WebAssembly and Blazor Server apps, the server-side default route template assumes that if the last segment of a request URL contains a dot (.
) that a file is requested. For example, the URL https://localhost.com:5001/example/some.thing
is interpreted by the router as a request for a file named some.thing
. Without additional configuration, an app returns a 404 - Not Found response if some.thing
was meant to route to a component with an @page
directive and some.thing
is a route parameter value. To use a route with one or more parameters that contain a dot, the app must configure the route with a custom template.
Consider the following Example
component that can receive a route parameter from the last segment of the URL.
Pages/Example.razor
:
To permit the Server
app of a hosted Blazor WebAssembly solution to route the request with a dot in the param
route parameter, add a fallback file route template with the optional parameter in Startup.Configure
.
Startup.cs
:
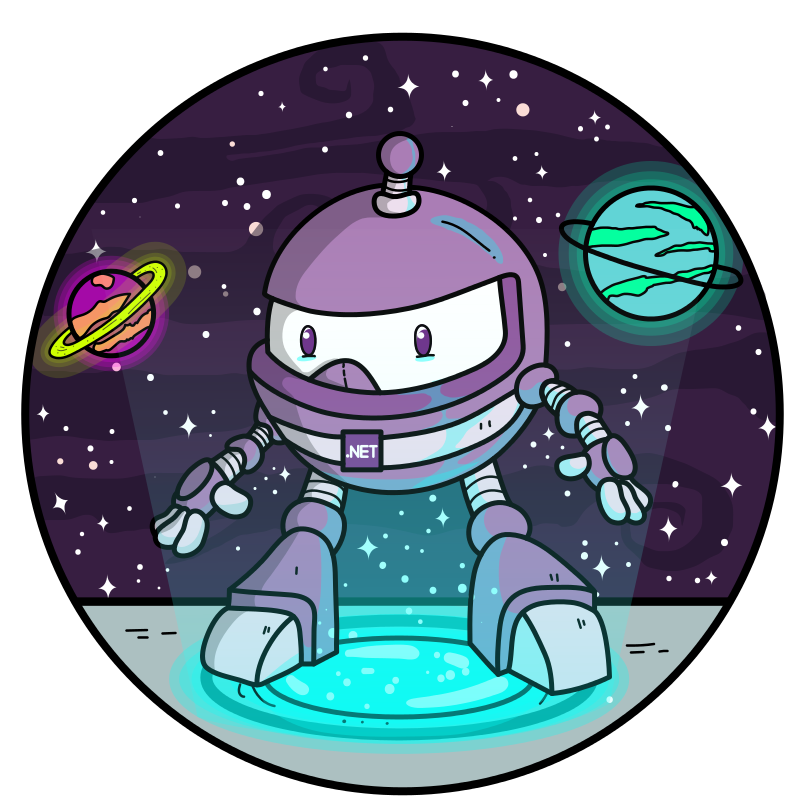
To configure a Blazor Server app to route the request with a dot in the param
route parameter, add a fallback page route template with the optional parameter in Startup.Configure
.
Startup.cs
:
Markdig Blazor
For more information, see Routing in ASP.NET Core.
Catch-all route parameters
Catch-all route parameters, which capture paths across multiple folder boundaries, are supported in components.
Catch-all route parameters are:
- Named to match the route segment name. Naming isn't case sensitive.
- A
string
type. The framework doesn't provide automatic casting. - At the end of the URL.
Pages/CatchAll.razor
:
For the URL /catch-all/this/is/a/test
with a route template of /catch-all/{*pageRoute}
, the value of PageRoute
is set to this/is/a/test
.
Slashes and segments of the captured path are decoded. For a route template of /catch-all/{*pageRoute}
, the URL /catch-all/this/is/a%2Ftest%2A
yields this/is/a/test*
.
Catch-all route parameters are supported in ASP.NET Core 5.0 or later. For more information, select the 5.0 version of this article.
URI and navigation state helpers
Use NavigationManager to manage URIs and navigation in C# code. NavigationManager provides the event and methods shown in the following table.
Member | Description |
---|---|
Uri | Gets the current absolute URI. |
BaseUri | Gets the base URI (with a trailing slash) that can be prepended to relative URI paths to produce an absolute URI. Typically, BaseUri corresponds to the href attribute on the document's <base> element in wwwroot/index.html (Blazor WebAssembly) or Pages/_Host.cshtml (Blazor Server). |
NavigateTo | Navigates to the specified URI. If forceLoad is true :
|
LocationChanged | An event that fires when the navigation location has changed. |
ToAbsoluteUri | Converts a relative URI into an absolute URI. |
ToBaseRelativePath | Given a base URI (for example, a URI previously returned by BaseUri), converts an absolute URI into a URI relative to the base URI prefix. |
For the LocationChanged event, LocationChangedEventArgs provides the following information about navigation events:
- Location: The URL of the new location.
- IsNavigationIntercepted: If
true
, Blazor intercepted the navigation from the browser. Iffalse
, NavigationManager.NavigateTo caused the navigation to occur.
The following component:
- Navigates to the app's
Counter
component (Pages/Counter.razor
) when the button is selected using NavigateTo. - Handles the location changed event by subscribing to NavigationManager.LocationChanged.
The
HandleLocationChanged
method is unhooked whenDispose
is called by the framework. Unhooking the method permits garbage collection of the component.The logger implementation logs the following information when the button is selected:
BlazorSample.Pages.Navigate: Information: URL of new location: https://localhost:5001/counter
Pages/Navigate.razor
:
For more information on component disposal, see ASP.NET Core Razor component lifecycle.
Query string and parse parameters
The query string of a request is obtained from the NavigationManager.Uri property:
To parse a query string's parameters, one approach is to use URLSearchParams
with JavaScript (JS) interop:
For more information, see Blazor JavaScript isolation and object references.
NavLink
and NavMenu
components
Use a NavLink component in place of HTML hyperlink elements (<a>
) when creating navigation links. A NavLink component behaves like an <a>
element, except it toggles an active
CSS class based on whether its href
matches the current URL. The active
class helps a user understand which page is the active page among the navigation links displayed. Optionally, assign a CSS class name to NavLink.ActiveClass to apply a custom CSS class to the rendered link when the current route matches the href
.
Blazor Markdown Viewer
The following NavMenu
component creates a Bootstrap
navigation bar that demonstrates how to use NavLink components:
Note
The NavMenu
component (NavMenu.razor
) is provided in the Shared
folder of an app generated from the Blazor project templates.
There are two NavLinkMatch options that you can assign to the Match
attribute of the <NavLink>
element:
- NavLinkMatch.All: The NavLink is active when it matches the entire current URL.
- NavLinkMatch.Prefix (default): The NavLink is active when it matches any prefix of the current URL.
In the preceding example, the Home NavLinkhref='
matches the home URL and only receives the active
CSS class at the app's default base path URL (for example, https://localhost:5001/
). The second NavLink receives the active
class when the user visits any URL with a component
prefix (for example, https://localhost:5001/component
and https://localhost:5001/component/another-segment
).
Additional NavLink component attributes are passed through to the rendered anchor tag. In the following example, the NavLink component includes the target
attribute:
The following HTML markup is rendered:
Blazor Markdown Control
Warning
Due to the way that Blazor renders child content, rendering NavLink
components inside a for
loop requires a local index variable if the incrementing loop variable is used in the NavLink
(child) component's content:
Using an index variable in this scenario is a requirement for any child component that uses a loop variable in its child content, not just the NavLink
component.
Alternatively, use a foreach
loop with Enumerable.Range:
ASP.NET Core endpoint routing integration
This section only applies to Blazor Server apps.
Blazor Server is integrated into ASP.NET Core Endpoint Routing. An ASP.NET Core app is configured to accept incoming connections for interactive components with MapBlazorHub in Startup.Configure
.
Startup.cs
:
The typical configuration is to route all requests to a Razor page, which acts as the host for the server-side part of the Blazor Server app. By convention, the host page is usually named _Host.cshtml
in the Pages
folder of the app.
The route specified in the host file is called a fallback route because it operates with a low priority in route matching. The fallback route is used when other routes don't match. This allows the app to use other controllers and pages without interfering with component routing in the Blazor Server app.
Blazor Markdown
For information on configuring MapFallbackToPage for non-root URL server hosting, see Host and deploy ASP.NET Core Blazor.
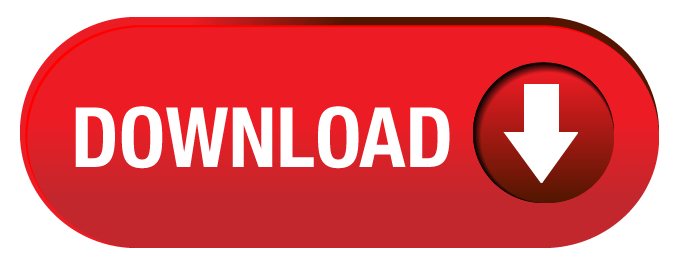